Introduction
The purpose of this document is to demonstrate the creation of
plugins for the Pandion instant messaging client. The reader of
this documentation should have a basic understanding of XML, XMPP and
the JScript programming language. Any UTF-8 compatible text editor
can be used to develop Pandion plug-ins.
What are plug-ins?
Plug-ins are tab sized websites, event driven JScript code, or a combination of both.
Plug-ins are encapsulated in a single XML document. This XML
document can contain meta information, script code, event handlers, or
other files.
Plug-in XML documents are extracted, installed and loaded when the client is started.
Example 1: This plug-in loads the BBC News website in a tab
<?xml version="1.0"?>
<plugin type="tab-iframe">
<name>BBC</name>
<description>
Stay informed with up-to-date news coverage.
</description>
<version>1.0</version>
<file>
<name>bbc.gif</name>
<data content="base64">
R0lGODlhGAAYAKIHAKmqqXFxcQUFBSkpKerq6v///wAAAP///yH5BAEAAAcALAAAAAAYABgA
AAOkeLrc/jAyEqq9GBNFhDCD8XkkOZSBAnxGK5ww285GenQ0QOw7cNItG4EmABSOyEJAFuSE
XEZKYEA4Pme21axY8LWoSqBQxsXVqj+s6ioIFAiAgE7JrHHIxuRRS8vi33EAc3xNN0RRJm5h
ageEbV0yYAF9HIdvLI9dlI1/PXNvV3Y3f3o7oaIARAMYA6eFQ0Cxsqittba3uDY3PLy9vjsS
wcLDCwkAOw==
</data>
</file>
<tab>
<icon>bbc.gif</icon>
<tooltip>BBC</tooltip>
</tab>
<event for="onLoad">
<url>
http://www.bbc.co.uk/mobile/index.html
</url>
</event>
</plugin>
|
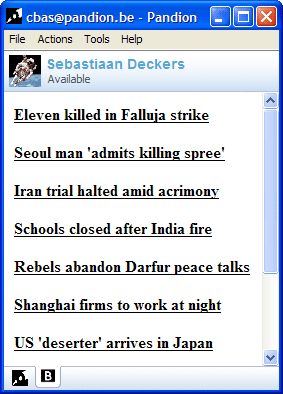 |
Types of Plug-ins
The type attribute of the root <plugin> element in the XML
document determines how the plug-in will be loaded and how events will
be handled. These are the possible values for type:
- tab-iframe
A tab will be created in the contact list window for this plug-in.
This tab is an <iframe/> in the DOM of src/main.html and is
initialized at about:blank. The src property of this iframe is
controlled by the values of the file and url tags in the event handlers.
- tab-html
A tab will be created in the contact list window for this plug-in.
The tab is a <div/> in the DOM of src/main.html. This
<div/> element is initialized with the data of the first
<html/> element in the plug-in XML file.
- code
No tab is created for this plug-in type. This is the
recommended type for plug-ins which either do not require to be
displayed or open custom windows.
Meta Information
The settings window displays all currently loaded plug-ins based on these values.
This data is required for plug-ins:
These tags are children of the root <plugin/> element.
Packaging and Installing Files
Plug-ins can contain extra data such as images, code files, libraries, media files, etc.
The <file/> element offers a generic and flexible way to
package these files. The data is either plain text inside a CDATA
structure or encoded as BASE64.
Files are extracted to their own unique directory. The name of
this directory is the SHA-1 hash of the plug-in XML filename.
The name of a packaged file is the data of the <name/>
element. This name can not include a subdirectory hierarchy.
The standard Windows filename conventions and limitations apply.
The filename is limited to 215 characters.
Example 2: The BASE64 encoded version of a GIF file and the extracted file
<file>
<name>bbc.gif</name>
<data content="base64">
R0lGODlhGAAYAKIHAKmqqXFxcQUFBSkpKerq6v///wAAAP///yH5BAEAAAcALAAAAAAYABgA
AAOkeLrc/jAyEqq9GBNFhDCD8XkkOZSBAnxGK5ww285GenQ0QOw7cNItG4EmABSOyEJAFuSE
XEZKYEA4Pme21axY8LWoSqBQxsXVqj+s6ioIFAiAgE7JrHHIxuRRS8vi33EAc3xNN0RRJm5h
ageEbV0yYAF9HIdvLI9dlI1/PXNvV3Y3f3o7oaIARAMYA6eFQ0Cxsqittba3uDY3PLy9vjsS
wcLDCwkAOw==
</data>
</file>
|
 |
Example 3: Plaintext HTML file inside a CDATA structure and the extracted file
<name>loading.html</name>
<data content="plain">
<![CDATA[
<html>
<body>
<br><br><br><br>
<center style="font-size: 11px; font-family: Tahoma;">Loading...</center>
</body>
</html>
]]>
</data>
</file>
|
<html>
<body>
<br><br><br><br>
<center style="font-size: 11px; font-family: Tahoma;">Loading...</center>
</body>
</html>
|
Tab Customization
Plug-ins of type tab-iframe or tab-html must display an icon and a tool tip.
Example 4: Tab button with a GIF icon and tool tip
<tab>
<icon>bbc.gif</icon>
<tooltip>BBC</tooltip>
</tab>
|
|
The icon must be a BMP, PNG, GIF, or JPEG image with a resolution of
16 pixels by 16 pixels. If the icon is larger or smaller than this
resolution in either height or width, it will be scaled. The
value of <icon/> is the filename of a packaged file in the same
plug-in XML file.
The <tooltip/> tag contains a text string. It is displayed when the user holds the mouse over the tab button.
Event Handling
Plug-ins can listen for events to execute code or navigate their
Iframe tab. Events related to XMPP have an optional
condition. The xpath parameter of the <event/> tag contains
an XPath expression which must be matched to fire the event.
Listing 1: Default events
onConnect |
Connection with the XMPP server is being established. |
onDisconnect |
Connection with the XMPP server was broken. |
onLoad |
The plug-in is loaded, usually when the client starts. |
onLoginFailure |
Can not log in to the XMPP server. Bad password, no network connection, server error, etc. |
onLoginSuccess |
Completely logged in to the XMPP server and retrieved the contact list. |
onMenu |
The user clicked a menu option or button (see below). |
onXMPPIQ |
<iq/> stanza has been received from the XMPP server matching the xpath attribute. |
onXMPPMessage |
<message/> stanza has been received from the XMPP server matching the xpath attribute. |
onXMPPPresence |
<presence/> stanza has been received from the XMPP server matching the xpath attribute. |
Multiple <event/> handlers may be set for the same event and
different actions such as <url/> and <code/> may be defined
for a single handler.
Listing 2: Actions that are supported by the event handler
<file/> |
Only for plug-ins of the type tab-iframe. The value of the
<file> tag is the name of a packaged file in the same plug-in XML
file. This file replaces the location of the tab’s Iframe.
|
<url/> |
Only for plug-ins of the type tab-iframe. The value of the
<url> tag is a HTTP address. This address replaces the location of
the tab’s Iframe. To facilitate authentication with web services, the
address may contain tokens such as login credentials of the XMPP user.
See below.
|
<code/> |
The <code> tag contains JScript code. The code is loaded into a
JScript Function object and the parameter attribute of the <code>
tag specifies the variable name of the function parameter. The function
parameter is a Dictionary object containing information about the
event. |
The <url/> address may contain tokens. These will be
replaced by the actual values when the event is fired. Use the
${…-sha1} and ${random} tokens to perform secure authentication.
Listing 3: URL tokens
${softwarename} |
The name of the IM client. Used for co-branding of the interface. |
${softwareversion} |
The version of the IM client. Used for co-branding of the interface. |
${username} |
The account identifier used to log in to the XMPP server. |
${server} |
The address of the XMPP server. |
${resource} |
The connection identifier used to log in to the XMPP server. |
${password} |
The secret key used to log in to the XMPP server. |
${random} |
A random floating point number. |
${username-sha1} |
SHA-1 hash of “${random}${username}” (without quotes). |
${server-sha1} |
SHA-1 hash of “${random}${server}” (without quotes). |
${resource-sha1} |
SHA-1 hash of “${random}${resource}” (without quotes). |
${password-sha1} |
SHA-1 hash of “${random}${password}” (without quotes). |
Menu Integration
The mouse menu of items in the contact list may be extended through
the use of <menu/> and <option/> tags. Clicking these
options fires the onMenu event. In future releases more menus and
controls will be made extensible.
Each menu extension displays a name and returns the corresponding
value. A single plug-in can have multiple extensions for the same
menu.
The onlineonly attribute specifies that this menu item should only be
enabled when the contact is online, and his address has a
resource. Contacts on XMPP transports generally do not have
resources.
Example 5: Extending the contact list mouse menu
<menu name="roster">
<option onlineonly="yes">
<text>Play a Game</text>
<value>play_game</value>
</option>
</menu>
|
|
Comments (0)
You don't have permission to comment on this page.